Building with Components
Learn how composition helps build complex objects by combining smaller components
Inheritance is useful, but sometimes we need more flexibility. This lesson introduces composition, a powerful design pattern where we build complex objects by combining smaller, focused "component" objects. We'll explore why inheritance isn't always the best fit and see how composition allows us to mix and match capabilities like animation, audio, and physics for different game entities.
As we build more complex projects, the objects we need to build become more and more powerful. Objects in a game, for example, might need capabilities including:
- The ability to be animated
- The ability to emit audio
- The ability to simulate physics and collide with other objects
A class that includes all of these capabilities would already be quite complex, and these represent only a tiny subset of the capabilities that objects need in more complex games.
Why Can't we use Inheritence?
We're already familiar with one way of managing the complexity in a class: we can organise these capabilities into a hierarchy, and then use inheritence to allow one class to include the capabilities of its parent.
However, inheritence isn't really sufficient here, as these abilities don't naturally exist in a hierarchy. Any given object will need some combination of these capabilities. For example:
- A wall needs to have physical collisions but doesn't need to be animated.
- A fire needs to be animated and emit audio but doesn't need to have physical collisions.
- An enemy monster needs all 3 of these capabilities, and more.
It's not possible to arrange these capabilities into an inheritence tree in an elegant way. We would end up with the classes being too complex - for example, a class containing audio-emitting capability that most of their objects do not require. Alternatively, we'd end up having duplication, such as the code to emit audio being written in multiple classes.
So, we need a better design to organise this.
Composition
Much like the event loop, composition is another example of a design pattern. It doesn't involve any new language capabilities - rather, it uses the techniques that we've already covered. It applies these techniques to build a system whose design has proven itself to be an effective way to solve a common problem we encounter when building complex software.
Composition is the simple idea of combining multiple objects to create more complex behaviours.
First, we break our required capabilites into dedicated classes, such as a class to manage audio, a class to manage animation, and so on. Objects we create from these classes are often referred to as components.
Then, we create another class that is designed to manage a collection of components. Instances of this second class are often called entities.
This creates a symbiotic system where:
- Components give capabilities to the entities they're attached to
- Entities decide what capabilities they need by choosing which types of components to attach
This gives us an elegant solution to our original problem. If an entity needs collision and audio capabilities, we can attach a PhysicsComponent
and an AudioComponent
to it. If some other entity needs animation and audio, it can have an AnimationComponent
and an AudioComponent
.
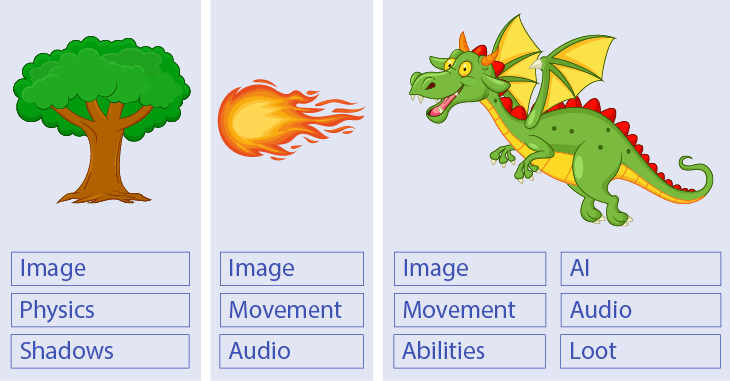
Creating entities by composing components
An Example of Composition
In the next lesson, we'll implement a flexible component system, where our entities can have any number of components, and where we can easily create new component types to grant our entities new capabilities.
For now, let's introduce a simple, minimalist example of composition to review some important concepts and establish the core idea. Feel free to skip this section if you're more comfortable - we'll be discarding the code we create here and begin building our more advanced system starting with the next lesson.
Base Classes
We'll start by creating a base class for all of our entities. It contains the usual Tick()
, HandleEvent()
, and Render()
functions for connecting to our game loop later:
// Entity.h
#pragma once
#include <SDL.h>
#include <string>
class Entity {
public:
Entity(std::string Name) : Name{Name} {}
std::string Name;
virtual void Tick(float DeltaTime) {}
virtual void HandleEvent(SDL_Event& E) {}
virtual void Render(SDL_Surface* S) {}
};
And, similarly, we'll create a base class for our components. They'll also contain the three methods that we need to connect our component to the game loop.
For a component to empower the entity it's attached to with some capability, it will typically need to know what that entity is. As such, we'll store a pointer to that Entity
, and initialize it from the constructor:
// Component.h
#pragma once
#include <SDL.h>
class Entity;
class Component {
public:
Component(Entity* Parent) : Parent{Parent} {}
virtual void Tick(float DeltaTime) {}
virtual void HandleEvent(SDL_Event& E) {}
virtual void Render(SDL_Surface* S) {}
protected:
Entity* Parent{nullptr};
};
Extending Base Classes
Let's extend these base classes with some specific component types that will confer appropriate abilities to the entity they're attached to. We'll simulate this with some simple logging in Tick()
overrides for now, but we'll create useful components later in the chapter:
// Component.h
#pragma once
#include <SDL.h>
#include <string>
#include <iostream>
#include "Entity.h"
class Component {/*...*/};
class AnimationComponent : public Component {
public:
using Component::Component;
void Tick(float DeltaTime) override;
};
class AudioComponent : public Component {
public:
using Component::Component;
void Tick(float DeltaTime) override;
};
class PhysicsComponent : public Component {
public:
using Component::Component;
void Tick(float DeltaTime) override;
};
// Component.cpp
#include <iostream>
#include "Component.h"
#include "Entity.h"
void AnimationComponent::Tick(float DeltaTime) {
std::cout << Parent->Name << ": Animating\n";
}
void AudioComponent::Tick(float DeltaTime) {
std::cout << Parent->Name << ": Audio\n";
}
void PhysicsComponent::Tick(float DeltaTime) {
std::cout << Parent->Name << ": Physics\n";
}
Our Entity
subtypes can now select the abilities they need by constructing appropriate components, and connecting them to the game loop by forwarding the required function calls.
We're only using Tick()
in the following example, but you can also override HandleEvent()
and Render()
if required.
For now, the specific Entity
subtype determines what components each instance will have. The system we build later in the chapter will be much more flexible but, for this lesson, we'll keep things simple:
// Entity.h
#pragma once
#include <SDL.h>
#include <string>
#include "Component.h"
class Entity {/*...*/};
class Wall : public Entity {
public:
// Inherit the Entity(std::string) constructor
using Entity::Entity;
void Tick(float DeltaTime) override {
Physics.Tick(DeltaTime);
}
private:
PhysicsComponent Physics{this};
};
class Fire : public Entity {
public:
using Entity::Entity;
void Tick(float DeltaTime) override {
Animation.Tick(DeltaTime);
Audio.Tick(DeltaTime);
}
private:
// Fire has Animation and Audio components
AnimationComponent Animation{this};
AudioComponent Audio{this};
};
class Monster : public Entity {
public:
using Entity::Entity;
void Tick(float DeltaTime) override {
Animation.Tick(DeltaTime);
Audio.Tick(DeltaTime);
Physics.Tick(DeltaTime);
}
private:
// Monster has all three components
AnimationComponent Animation{this};
AudioComponent Audio{this};
PhysicsComponent Physics{this};
};
Connecting Everything Together
Let's create our basic Scene
class to construct and manage some entities:
// Scene.h
#pragma once
#include <SDL.h>
#include <vector>
#include <memory> // for std::unique_ptr, std::make_unique
#include "Entity.h" // for Wall, Fire, Monster
// Type aliases for clarity
using EntityPtr = std::unique_ptr<Entity>;
using EntityPtrs = std::vector<EntityPtr>;
class Scene {
public:
Scene() {
// Create entities, passing required names
// to constructors
Entities.emplace_back(
std::make_unique<Wall>("Wall_1"));
Entities.emplace_back(
std::make_unique<Fire>("Fire_1"));
Entities.emplace_back(
std::make_unique<Monster>("Monster_1"));
}
void HandleEvent(SDL_Event& E) {
// Forward event handling to all entities
for (EntityPtr& Entity : Entities) {
Entity->HandleEvent(E);
}
}
void Tick(float DeltaTime) {
// Forward ticking to all entities
for (EntityPtr& Entity : Entities) {
Entity->Tick(DeltaTime);
}
}
void Render(SDL_Surface* Surface) {
// Forward rendering to all entities
for (EntityPtr& Entity : Entities) {
Entity->Render(Surface);
}
}
private:
EntityPtrs Entities;
};
Finally, let's hook everything up to a basic game loop. The following main.cpp
and Window.h
classes have not changed since the previous chapter:
Running our code, we should now see our various components interacting with the entities they are attached to, identified by their names:
Wall_1: Physics
Fire_1: Animating
Fire_1: Audio
Monster_1: Animating
Monster_1: Audio
Monster_1: Physics
Advanced Examples
Creating an entity and component system gives us a great deal of flexibility. It allows us to do things that are far beyond what can be achieved by inheritance alone.
The following image is from Unreal Engine, showing their implementation of how an entity can be constructed by composing a flexible hierarchy of components:
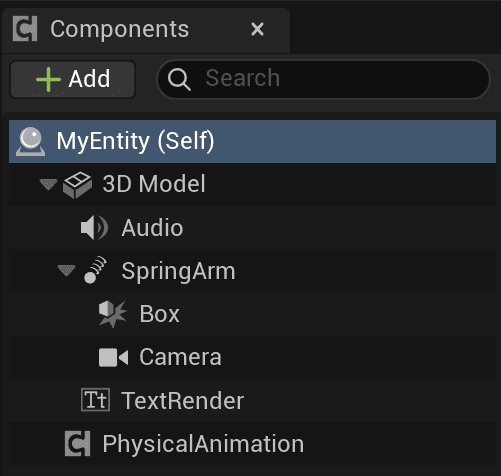
Creating an entity using components in Unreal Engine 5
By building a compositional framework, we can do things like:
- Control what components an entity has, independent of that entity's subtype. For example, one
Monster
might have a completely different set of components to anotherMonster
. - Attach multiple components of the same type. For example, if our entity needed to render two (or more) images, we don't need a class that can render multiple images - we can simply attach multiple image components to the entity.
- Add and remove components at run-time. This means our entities can gain or lose capabilities while our game is running, perhaps in response to player actions
- Attach components to other components, thereby creating a hierarchy. For example, this could allow our player entity to attach a component that renders a 3D model of a radio. We could then attach an audio component to that 3D model component, to emit sound from the location of the radio.
We'll implement these capabilities throughout the rest of this chapter.
Summary
This lesson introduced the composition design pattern as a flexible alternative to inheritance for building complex objects. We saw how entities can gain capabilities by attaching specialized components. While our initial example was simple, it demonstrated the core concept of combining objects to achieve greater flexibility than inheritance hierarchies typically allow.
- Inheritance struggles when capabilities don't fit a neat hierarchy.
- Composition builds complex objects by combining simpler objects (components).
- An entity manages a collection of components.
- Components provide specific capabilities (e.g., animation, physics) to their entity.
- Composition allows mixing and matching capabilities easily.
Implementing a Component System
Create the C++ framework for adding, retrieving, and removing components from game entities.